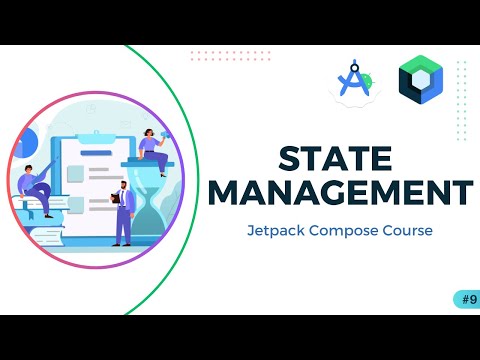
State Management in Jetpack Compose – Jetpack Compose Course #8
Video by Master Coding via YouTube
Source
πππ Check out my Udemy courses here:
π The Complete Android 15 Course [Part 1]-Master Java & Kotlin
https://www.udemy.com/course/the-complete-android-10-developer-course-mastering-android/?couponCode=MASTERCODING4
π The Complete Android 15 Course [Part 2] – Jetpack Compose
https://www.udemy.com/course/android15-developer-course-part2/?couponCode=MASTERCODING4
πππ What Youβll Learn in This Video ππ
ππ State management in Jetpack Compose is crucial for creating responsive and interactive UI components. Unlike the traditional View-based system, Jetpack Compose follows a declarative UI paradigm, meaning the UI is rebuilt in response to state changes rather than being modified imperatively.
1οΈβ£ Remember API
Jetpack Compose provides the remember function to retain state across recompositions:
var count by remember { mutableStateOf(0) }
remember {} ensures the state is retained across recompositions.
mutableStateOf(0) creates a mutable state that triggers recomposition when changed.
2οΈβ£ rememberSaveable for Persistence
If you want to persist state across configuration changes (e.g., screen rotation), use rememberSaveable:
var count by rememberSaveable { mutableStateOf(0) }
This works with types that support Bundle serialization.
3οΈβ£ State Hoisting (Lifting State Up)
Instead of managing state within a composable, it’s a best practice to lift state up to the parent:
@Composable
fun Counter(count: Int, onIncrement: () — Unit) {
Button(onClick = onIncrement) {
Text("Count: $count")
}
}
@Composable
fun CounterScreen() {
var count by remember { mutableStateOf(0) }
Counter(count) { count++ }
}
4οΈβ£ ViewModel for State Management
For long-lived state, such as data fetching from Firestore, use ViewModel:
class CounterViewModel : ViewModel() {
private val _count = MutableStateFlow(0) // StateFlow for Compose compatibility
val count: StateFlow (Int) = _count
fun increment() {
_count.value++
}
}
@Composable
fun CounterScreen(viewModel: CounterViewModel = viewModel()) {
val count by viewModel.count.collectAsState()
Counter(count, onIncrement = { viewModel.increment() })
}
StateFlow (or LiveData) ensures state survives process death and recompositions.
5οΈβ£ DerivedStateOf for Performance Optimization
If a state depends on another state, use derivedStateOf to avoid unnecessary recompositions:
val isEven by remember { derivedStateOf { count % 2 == 0 } }
ππ Donβt just watchβtake action! Start your journey today and join thousands of students whoβve transformed their careers with my courses. Letβs build something amazing together!
π If you found this video helpful, please give it a thumbs up, share it with your friends, and subscribe to my channel for more tutorials like this!
π Turn on the notification bell so you never miss an update.
#AndroidStudio #AndroidDevelopment #LearnToCode #UdemyCourses #AppDevelopment #ProgrammingTutorial